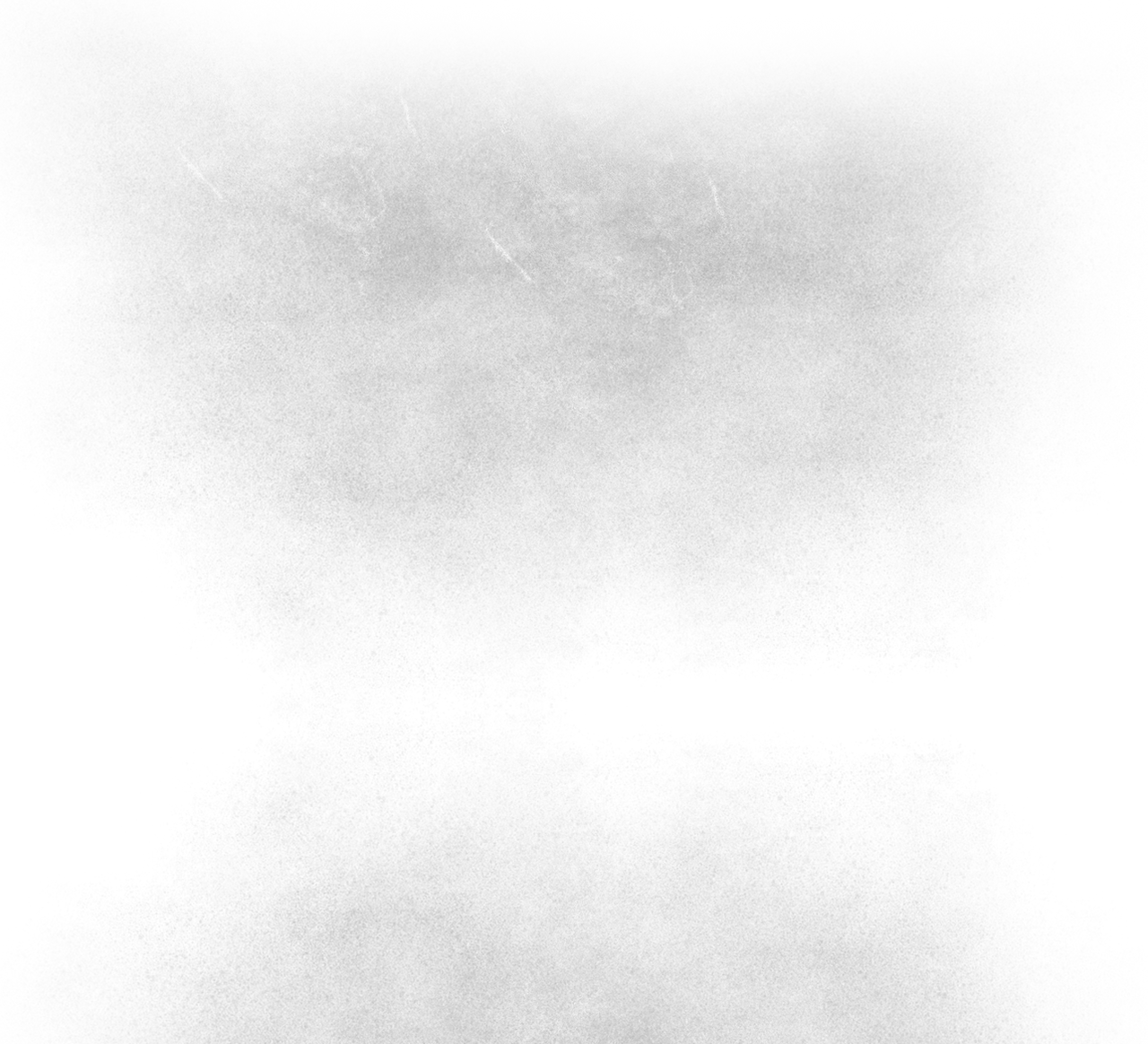

AnterakZero
Games Developer

Microsoft Advertising SDK running on Unity without store plugins
!!!! Microsoft Advertising SDK is now unavailable !!!!
1. Download Microsoft Advertising SDK here.
2. Open Visual Studio and create a new Windows Runtime Component (UWP) project called WindowsAdsComponent.
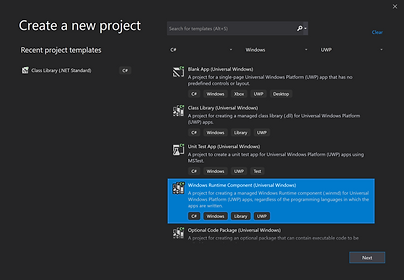
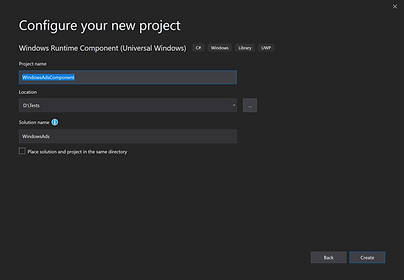
3. Add a Microsoft Advertising SDK reference to your project as below.
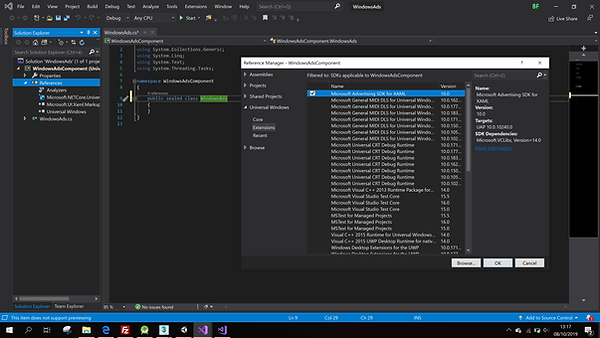
​
4. Set your solution configuration to Release and platform to x64.
5. Copy the following code into your WindowsAds.cs file. (Copy the code from the Word link.)
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
// Don't forget to add this 3 namespaces !
using Microsoft.Advertising.WinRT.UI;
using Windows.ApplicationModel.Core;
using Windows.UI.Core;
​
namespace WindowsAdsComponent
{
public enum ShowResult
{
Ready,
Error,
Completed,
Cancelled
}
​
public sealed class WindowsAds
{
private bool mAdsInit = false;
private bool mExceptionGenerate = false;
private string myAppId = "d25517cb-12d4-4699-8bdc-52040c712cab"; //Test spot ads string;
private string myAdUnitId = "test";
private ShowResult mShowResult;
private InterstitialAd myInterstitialAd = null;
​
// Events
public event EventHandler<ShowResult> videoShow;
​
// Properties
public bool AdsInit
{
get { return mAdsInit; }
set { mAdsInit = value; }
}
public bool ExceptionGenerate
{
get { return mExceptionGenerate; }
set { mExceptionGenerate = value; }
}
public string AppId
{
get { return myAppId; }
set { myAppId = value; }
}
public string AdUnitId
{
get { return myAdUnitId; }
set { myAdUnitId = value; }
}
private ShowResult showResult
{
get { return mShowResult; }
set
{
mShowResult = value;
EventHandler<ShowResult> handler = videoShow;
if (handler != null)
{
handler(this, mShowResult);
}
}
}
​
// constructor
public WindowsAds()
{
var t = CoreApplication.MainView.CoreWindow.Dispatcher.RunAsync(
CoreDispatcherPriority.Normal, () => {
try
{
myInterstitialAd = new InterstitialAd();
myInterstitialAd.AdReady += MyInterstitialAd_AdReady;
myInterstitialAd.ErrorOccurred += MyInterstitialAd_ErrorOccurred;
myInterstitialAd.Completed += MyInterstitialAd_Completed;
myInterstitialAd.Cancelled += MyInterstitialAd_Cancelled;
mAdsInit = true;
mExceptionGenerate = false;
}
catch
{
mExceptionGenerate = true;
}
});
}
​
// request and show a video
public void ShowVideo()
{
if (!mExceptionGenerate && mAdsInit)
{
var t = CoreApplication.MainView.CoreWindow.Dispatcher.RunAsync(
CoreDispatcherPriority.Normal, () =>
{
try
{
myInterstitialAd.RequestAd(AdType.Video, myAppId, myAdUnitId);
mExceptionGenerate = false;
}
catch
{
mExceptionGenerate = true;
}
});
}
}
​
private void MyInterstitialAd_AdReady(object sender, object e)
{
showResult = ShowResult.Ready;
var t = CoreApplication.MainView.CoreWindow.Dispatcher.RunAsync(
CoreDispatcherPriority.Normal, () => {
try
{
myInterstitialAd.Show();
mExceptionGenerate = false;
}
catch
{
mExceptionGenerate = true;
}
});
}
​
private void MyInterstitialAd_ErrorOccurred(object sender, AdErrorEventArgs e)
{
showResult = ShowResult.Error;
}
private void MyInterstitialAd_Completed(object sender, object e)
{
showResult = ShowResult.Completed;
}
private void MyInterstitialAd_Cancelled(object sender, object e)
{
showResult = ShowResult.Cancelled;
}
}
}
6. Build the solution.
7. copy the WindowsAdsComponent.winmd file from ...\WindowsAds\WindowsAdsComponent\bin\x64\Release directory to your Asset\Plugins\WSA Unity project directory.
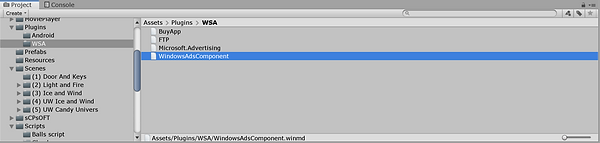
8. In your Unity game project, create a C# script called "AdsScript", and copy the following code.
​
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
​
#if WINDOWS_UWP
using WindowsAdsComponent;
#endif
​
public class AdsScript : MonoBehaviour
{
#if WINDOWS_UWP
private WindowsAds mWindowsAds;
private bool mbUnityThread = false; //used to send the event to Unity main thread.
private ShowResult showResult;
public delegate void VideoShow(WindowsAdsComponent.ShowResult showResult);
public event VideoShow onVideoShow;
#endif
​
// Start is called before the first frame update
void Start()
{
#if WINDOWS_UWP
mWindowsAds = new WindowsAds();
//mWindowsAds.AppId = fill with your store App ID string here
//mWindowsAds.AdUnitId = fill with your store Ads Unit ID string here
mWindowsAds.videoShow += OnVideoShow;
#endif
}
​
public void ShowRewardedVideo()
{
#if (UNITY_IOS || UNITY_ANDROID)
#endif
#if WINDOWS_UWP
mWindowsAds.ShowVideo();
#endif
}
​
#if WINDOWS_UWP
private void OnVideoShow(object sender, ShowResult e)
{
mbUnityThread = true;
showResult = e;
}
​
// Update is called once per frame
void Update()
{
if (onVideoShow != null && mbUnityThread)
{
mbUnityThread = false;
onVideoShow(showResult);
}
}
#endif
}
9. Now you can use the following code anywhere in a C# script associated to a gameobject item like gem or anything else.
​
​
AdsScrip ads = FindObjectOfType<AdsScript>();
ads.onVideoShow += ads_onVideoShow;
//Make a request to show a video ad.
ads.ShowRewardedVideo();
//Events from Microsoft ads server
#if WINDOWS_UWP
private void mAds_onVideoShow(WindowsAdsComponent.ShowResult showResult)
{
if (showResult == WindowsAdsComponent.ShowResult.Completed)
{
//the ad was fully viewed
}
if (showResult == WindowsAdsComponent.ShowResult.Cancelled)
{
//the ad was cancelled by the player
}
if (showResult == WindowsAdsComponent.ShowResult.Ready)
{
//the ad is ready to be shown
}
if (showResult == WindowsAdsComponent.ShowResult.Error)
{
//an errors has occured
}
}
#endif
​
10. Export your project to UWP.

10. !!THE MOST IMPORTANT!!
Open your UWP project with Visual Studio and add a Microsoft advertising SDK reference.
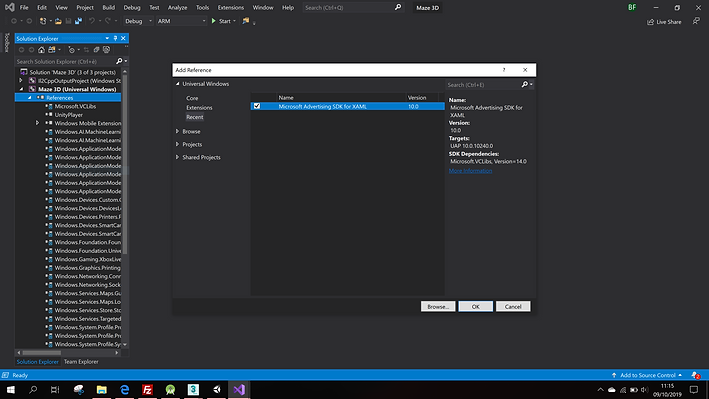